Problem
Managed Redis instances are expensive. But Redis is amazing, I use it for handling cache, sessions and queues.
When speaking to the AWS team about ways of cutting our bills down they recommended moving to DynamoDB and SQS, my initial question was:
“How do I run the same stuff locally when developing so im not incurring costs?”
Their answer was:
“Just run it against a real instance?” - ¯_(ツ)_/¯
I’m really, really big on being able to run things locally. So I set out on working out how I could do the above.
Continue reading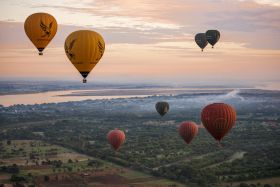